Foundations of Algorithms
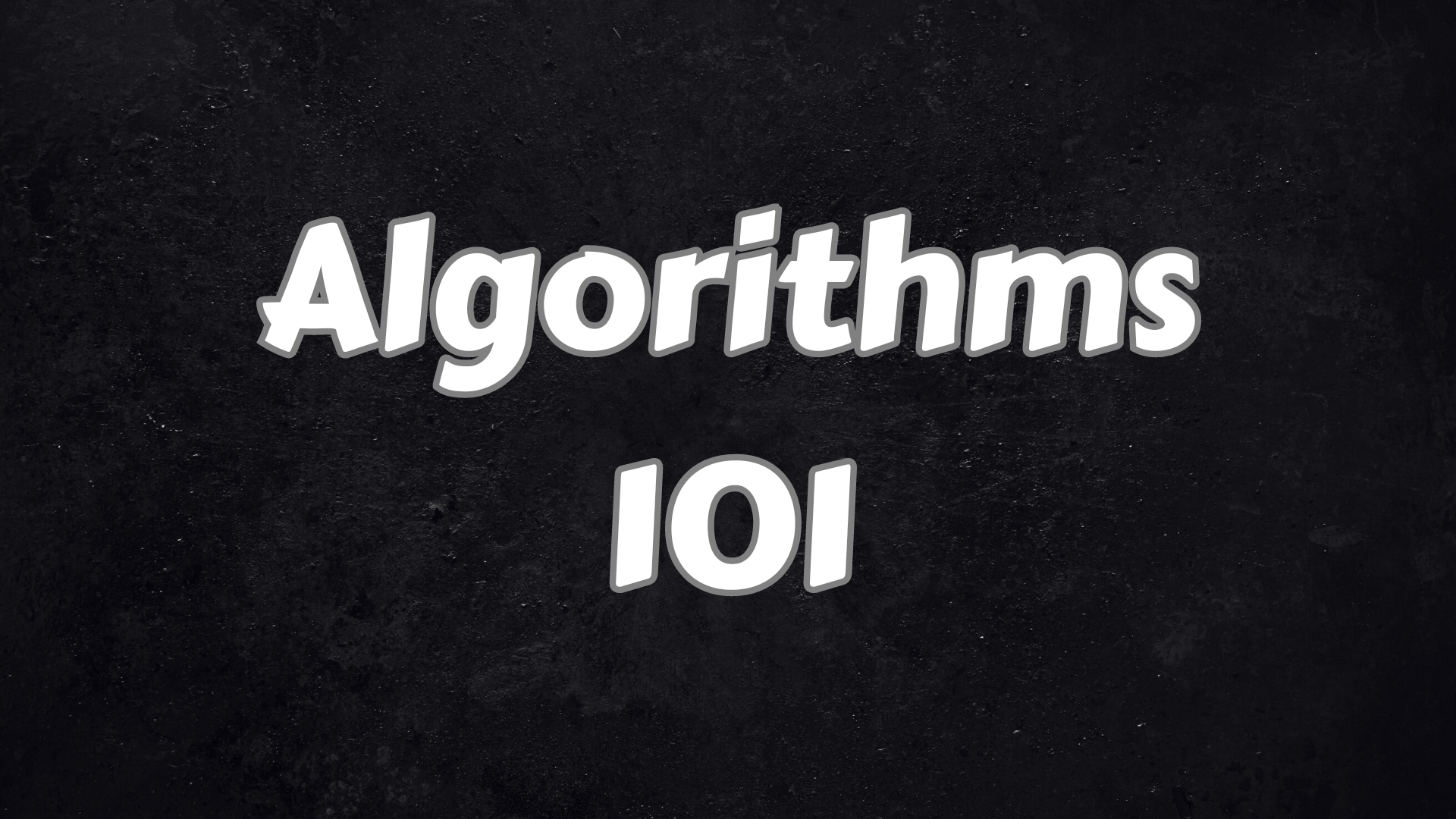
About Course
Welcome to our Algorithm 101 course! Algorithms are the backbone of computer science, empowering us to solve complex problems efficiently. This course is designed for beginners eager to dive into the world of algorithms, offering a comprehensive understanding of their significance and practical applications.
Course Outline:
1. Introduction to Algorithms
- Understanding the role of algorithms in problem-solving
- Types of algorithms and their applications
2. Fundamentals of Algorithm Analysis
- Big O notation and algorithm complexity
- Time and space complexity analysis
3. Sorting and Searching Algorithms
- Bubble sort, insertion sort, merge sort, and quicksort
- Linear and binary search algorithms
4. Data Structures
- Arrays, linked lists, stacks, queues, and trees
- How data structures influence algorithm design
5. Greedy Algorithms and Dynamic Programming
- Concepts of greedy algorithms
- Principles of dynamic programming
6. Graph Algorithms
- Depth-first search (DFS) and breadth-first search (BFS)
- Shortest path algorithms like Dijkstra’s and Bellman-Ford
7. Algorithm Design Techniques
- Divide and conquer, backtracking, and more
- Applying different strategies to solve problems effectively
8. Advanced Topics and Practical Applications
- Advanced algorithms: NP-hard problems, approximation algorithms
- Real-world applications in various fields
Course Format:
- Engaging video lectures
- Hands-on coding exercises and projects
- Quizzes and assessments to reinforce learning
- Discussion forums for collaboration and problem-solving
By the end of this course, you’ll have a solid foundation in algorithms, enabling you to approach problem-solving challenges with confidence and efficiency.
Join us on this exciting journey into the world of algorithms!
Course Content
Introduction to Algorithms
-
Understanding the role of algorithms in problem-solving
00:00 -
Types of algorithms and their applications
00:00