Object Oriented Programming For Beginners
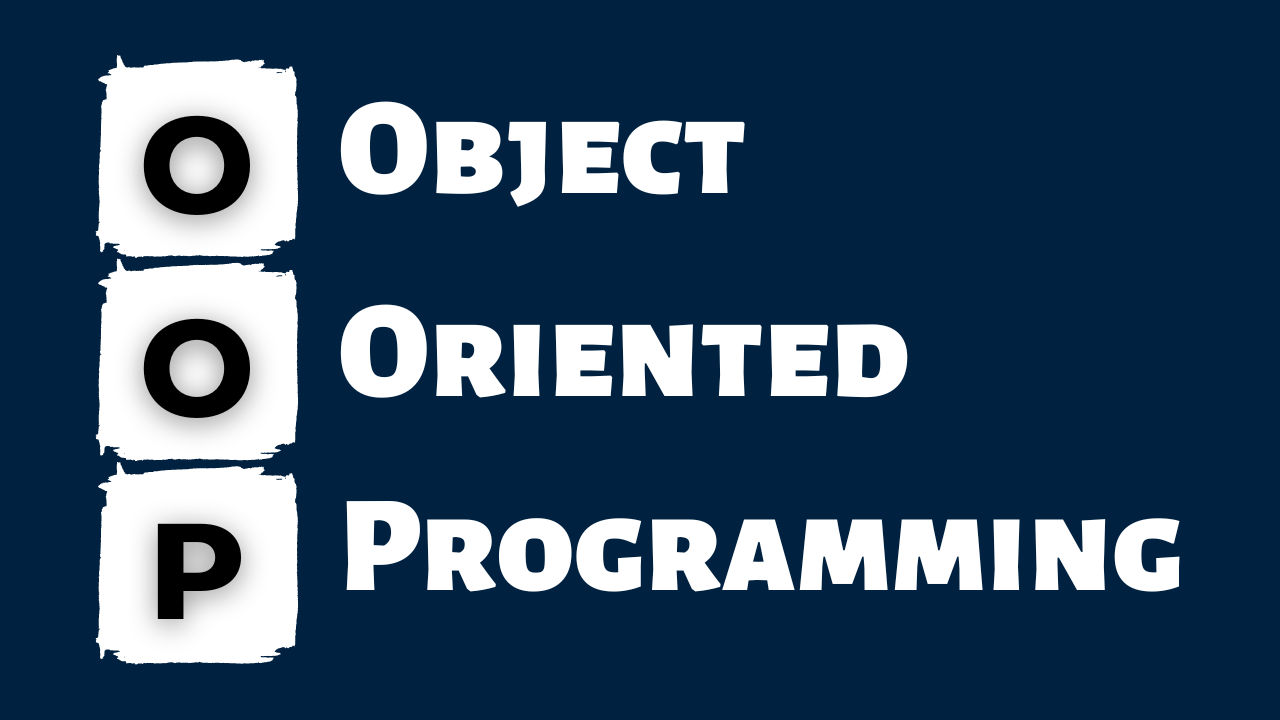
About Course
Welcome to the exciting world of Object-Oriented Programming (OOP)! This course is designed to introduce beginners to the fundamental concepts and principles of OOP, empowering them to build robust and efficient software using this widely adopted programming paradigm.
In this course, we will delve into the core concepts of OOP step by step, making the learning process accessible and engaging for all participants. Whether you are new to programming or seeking to enhance your coding skills, this course provides a solid foundation for understanding the principles that drive modern software development.
Course Outline:
- Introduction to Object-Oriented Programming:
- Understanding the evolution of programming paradigms
- Advantages of OOP and its real-world applications
- Objects and Classes:
- Defining objects and classes
- Properties and methods: Encapsulation of data and behavior
- Constructors and destructors: Initializing and cleaning up objects
- Inheritance:
- Creating hierarchies of classes
- Extending and inheriting behavior from parent classes
- Overriding methods and implementing polymorphism
- Encapsulation and Abstraction:
- Access modifiers: Public, private, and protected
- Abstraction: Hiding complexity and exposing essential features
- Polymorphism and Interfaces:
- Understanding interfaces and their role in OOP
- Achieving flexibility through polymorphism
- Interface implementation and multiple inheritance
- Object Relationships:
- Association, aggregation, and composition
- Navigating object relationships
- Building robust and maintainable software through proper design
- Exception Handling:
- Handling errors and unexpected situations gracefully
- Try-catch blocks and error propagation
- Design Patterns:
- Exploring common OOP design patterns
- Singleton, Factory, Observer, and more
- Applying patterns to solve real-world programming challenges
- OOP Best Practices:
- Writing clean and modular code
- Code reusability and maintainability
- Tips for effective OOP development
- Practical Projects:
- Building small-scale applications to apply the concepts learned
- Working collaboratively and integrating OOP principles into project development
By the end of this course, students will have gained a solid understanding of OOP concepts, enabling them to confidently create well-structured, scalable, and maintainable software solutions. Emphasis will be placed on hands-on learning and practical projects to ensure students can immediately apply their newfound skills in real-world scenarios.
Join us on this journey to unlock the power of Object-Oriented Programming and become a more proficient and effective software developer!
Course Content
Introduction to Object-Oriented Programming
-
Understanding the evolution of programming paradigms
00:00 -
Advantages of OOP and its real-world applications
00:00